微服务基本组成
Spring Cloud Stream
屏蔽底层消息中间件的差异,降低切换成本,统一消息的编程模型
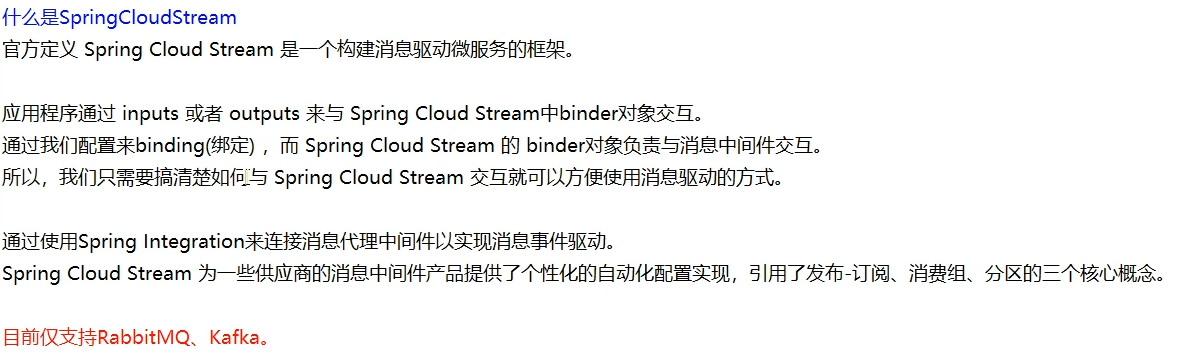
bander
通过定义绑定器Binder作为中间层,实现了应用程序与消息中间件细节之间的隔离
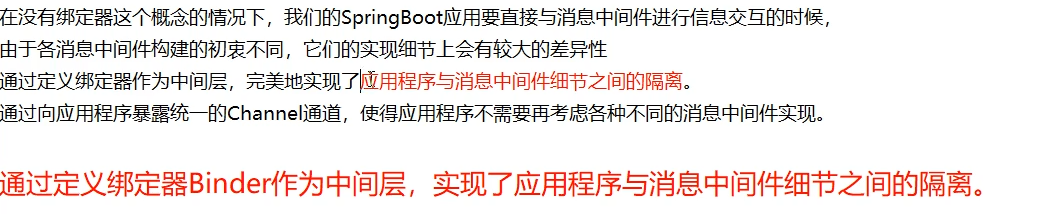
常用注解
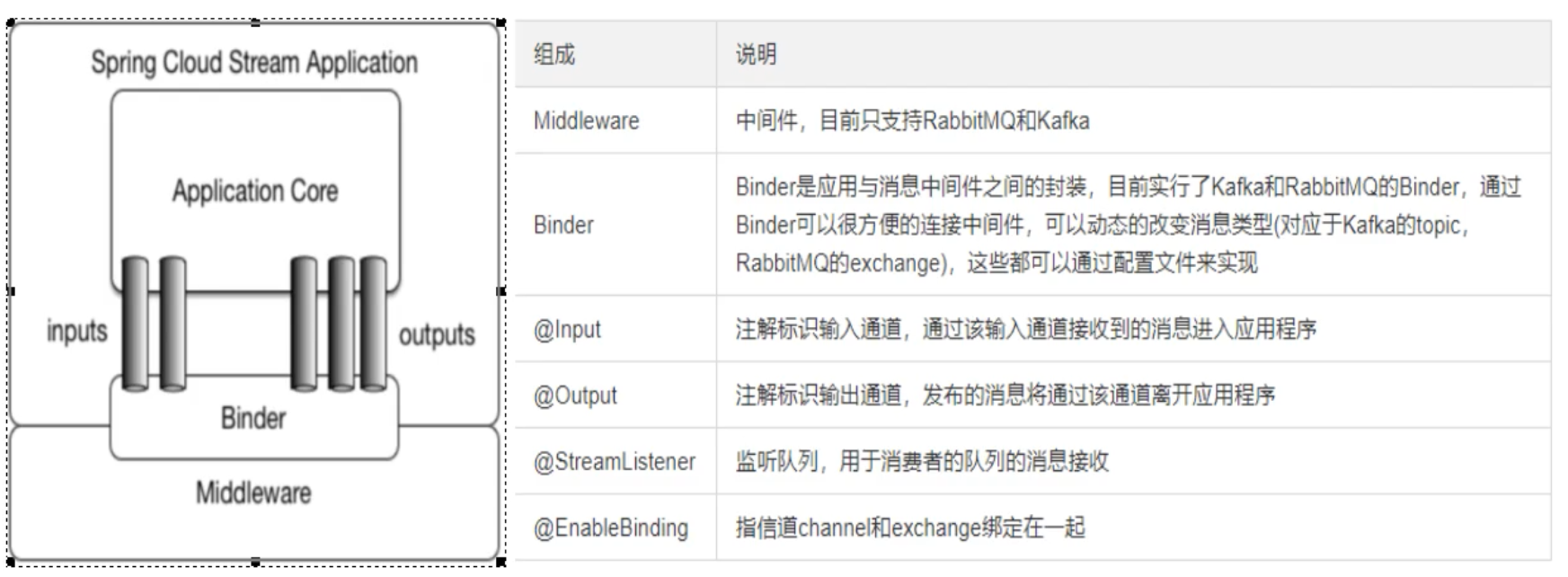
消息分组
微服务应用放置于同一个group中,就能保证消息只会被其中一个应用消费一次
不同的组是可以消费的,同一个组内会发生竞争关系,只有其中一个会被消费
可以避免消息丢失
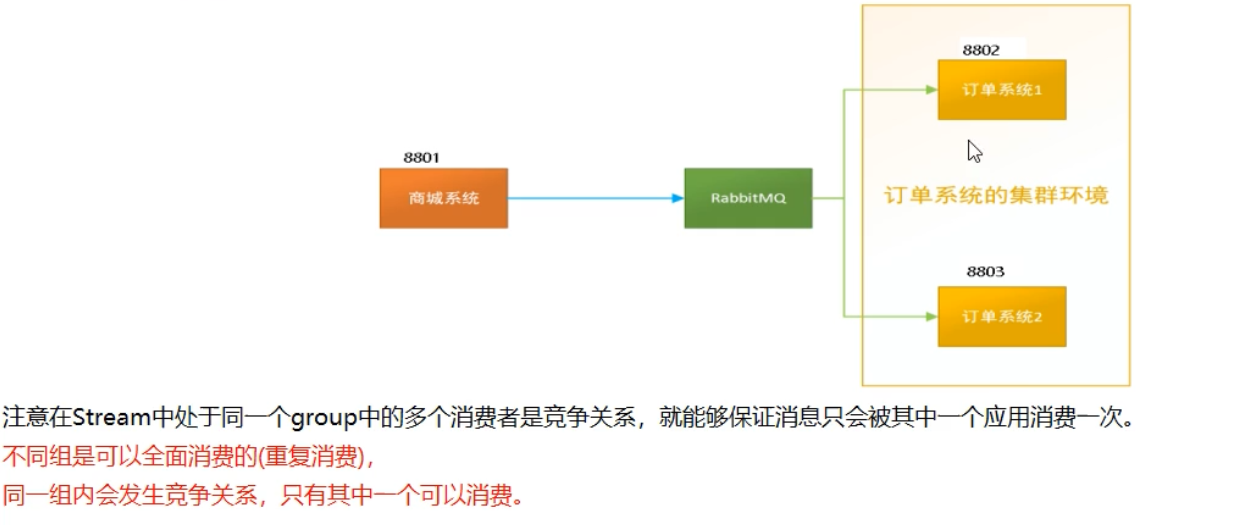
使用
生产者
pom
1 2 3 4 5
| <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-stream-rabbit</artifactId> </dependency>
|
yml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| server: port: 8801
spring: application: name: cloud-stream-provider cloud: stream: binders: defaultRabbit: type: rabbit environment: spring: rabbitmq: host: localhost port: 5672 username: guest password: guest bindings: output: destination: studyExchange content-type: application/json binder: defaultRabbit
eureka: client: service-url: defaultZone: http://localhost:7001/eureka instance: lease-renewal-interval-in-seconds: 2 lease-expiration-duration-in-seconds: 5 instance-id: send-8801.com prefer-ip-address: true
|
service
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public interface IMessageProvider { String send(); }
import com.atguigu.springcloud.service.IMessageProvider; import org.springframework.cloud.stream.annotation.EnableBinding; import org.springframework.messaging.MessageChannel; import org.springframework.integration.support.MessageBuilder;
import javax.annotation.Resource;
import org.springframework.cloud.stream.messaging.Source;
import java.util.UUID; @EnableBinding(Source.class) public class MessageProviderImpl implements IMessageProvider { @Resource private MessageChannel output;
@Override public String send() { String serial = UUID.randomUUID().toString(); output.send(MessageBuilder.withPayload(serial).build()); System.out.println("*****serial: "+serial); return null; } }
|
controller
1 2 3 4 5 6 7 8 9 10 11 12
| @RestController public class SendMessageController {
@Resource private IMessageProvider messageProvider;
@GetMapping(value = "/sendMessage") public String sendMessage(){ return messageProvider.send(); }
}
|
消费者
pom
1 2 3 4
| <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-stream-rabbit</artifactId> </dependency>
|
yml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| server: port: 8803
spring: application: name: cloud-stream-consumer cloud: stream: binders: defaultRabbit: type: rabbit environment: spring: rabbitmq: host: localhost port: 5672 username: guest password: guest bindings: input: destination: studyExchange content-type: application/json binder: defaultRabbit group: atguiguA
eureka: client: service-url: defaultZone: http://localhost:7001/eureka instance: lease-renewal-interval-in-seconds: 2 lease-expiration-duration-in-seconds: 5 instance-id: receive-8803.com prefer-ip-address: true
|
controller
1 2 3 4 5 6 7 8 9 10 11 12
| import org.springframework.beans.factory.annotation.Value; import org.springframework.cloud.stream.annotation.EnableBinding; import org.springframework.cloud.stream.annotation.StreamListener; import org.springframework.cloud.stream.messaging.Sink; import org.springframework.messaging.Message; import org.springframework.stereotype.Component; @StreamListener(Sink.INPUT) public void input(Message<String> message) { System.out.println("消费者2号,----->接受到的消息: "+message.getPayload()); }
|
相关文章
SpringCloud
服务注册与发现
SpringCloud-OpenFeign问题
SpringCloud-GateWay工具类
DockerCompose常用软件配置
SpringQuartz动态定时任务
Redis集群搭建
redis分布式锁
服务链路追踪
K8S